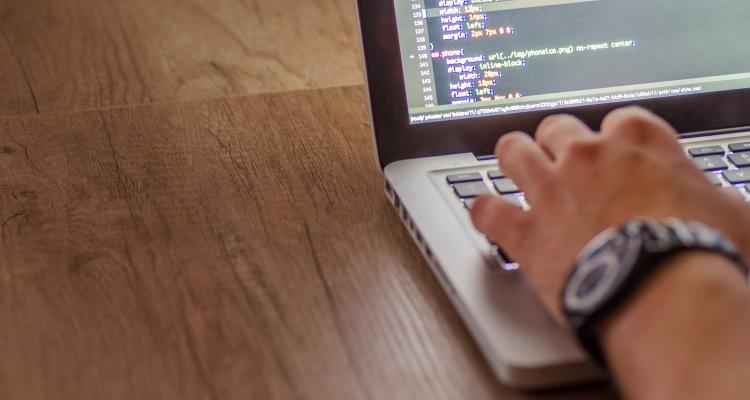
10 Practical Rules for Cleaner Code
May 9, 2018
This article is for my nerd friends. It is for software engineers that look for inspiration to write cleaner code.
Clean code is simple. Clean code is easy to modify. Clean code has few bugs. Clean code requires little work and achieves a better result.
Work smart - write clean code.
"Everything should be made as simple as possible - but not simpler."
1) Have conventions for code formatting and naming.
Do all your method and variable names start with lower case? Do all your class names start with upper case? For compound words, do you use camelCase? In method declarations, do you open the curly braces in a new line?
You want to have a set of rules how to format and name things. Clean code minimizes complexity. If there is inconsistent naming, you increase code complexity. In dirty code, you have to look up names each time you want to reuse code. Clean code follows conventions. Always.
2) Write out everything. Do not use abbreviations.
Software Developers don't spend most of the time typing code. They spend most of the time reading it. Clean code is easy to read.
You never want to use abbreviations to save a few seconds of typing. Instead, you want your code to be easy to understand. If you have a method that subtracts a discount from a price, don't call it subtrDisc($p). Instead, call it subtractDiscountFromPrice($price).
3) Name variables according to the company's terminology.
Each company develops its own terminology. For example, a high-value customer might be called Premier Customer. An inbound customer request that still needs handling might be called a support ticket.
When writing code, use the company's terminology. Don't name a variable $highValueCustomer when your company calls those people Premier Customers. By using the company's terminology, you have a clear understanding of the code's intentions - even after many years have passed.
4) Use short methods instead of comments.
A lot of fresh developers think that commented code is good code. I disagree. Comments are like dead code - they rot and nobody updates them. Comments get outdated as the code base changes. Over time, many comments become misleading rather than helpful.
If you have a block of code that is encapsulated in one logical concept - don't write a comment at the top. Instead, extract another short method. Most IDEs have shortcuts for method extraction - for example, CTRL+ALT+M in JetBrains' IDEs. The method name should be inspired by the comment you wanted to write. This way, you increase code reusability and readability.
5) Have a precise and consistent file and folder structure.
If your code is dirty, you use "Find Everywhere" in your IDE to search for the place you need to modify. If your code is clean and has a consistent structure, you can tell the location of every logic by browsing through the files. The location of a logic should be predictable. If you apply discounts, have a DiscountCalculator Class. Don't put the logic somewhere hidden in a Controller Class, or in a ShoppingCart Class or in a Checkout Class.
6) Use the same naming throughout every layer. Data -> Manipulation -> Presentation.
Giving the same thing multiple names is like opening Pandora's box of unnecessary complexity. You will flip between files to recall what synonyms have been used at what point in the code. Don't be the person that does the following: Your table is called "accounts"; the related DTO/Model is called "Customer"; the handling controller is the UsersController; you show the data at "https://www.domain.com/my-portal" which renders a view file called "mySettings.html" and uses a dedicated styling sheet called clientSettings.css with a javascript file called customerScripts.js.
Name it all the same. How does your business call them? Buyers? Then use that terminology and call everything related to the word buyer - from data (table) to manipulation (controllers, models, DTOs, components, services, ...) to presentation (endpoints, views, assets, css pointers, javascript functions, ...).
7) Separate responsibilities. Create dedicated classes.
Clean code can be understood at a high level by looking at nothing else but the files. When opening a file, its content should be predictable - there should be no element of surprise. The files lay out the details of what has been described by the file name.
In an InvoiceService, you shouldn't have a method called sendSms(). Nobody - including the future you in a few months - expects to find Sms related logic in an InvoiceService. Create your dedicated SmsService - even if it only contains one method. Think very hard where each piece of logic belongs - that's the only way to keep software modular and avoid spaghetti code.
8) Don't mix abstraction concepts with its implementation.
Clean code makes a clear separation between abstract ideas and concrete implementation. When you send out emails and you prepare related information, do it in an EmailComponent Class. If you use Mailgun to execute the actual sending, have a MailGun Class that implements the EmailInterface. Never have any MailGun specific logic in the abstract EmailService - otherwise you create coupling with third-parties. The business very often requires to interchange third-party APIs - your code needs to be prepared accordingly.
9) Use hierarchical translation keys.
Translation files consist of key -> value pairs. Use a consistent hierarchy to indicate the position of the item that needs translation. Ideally, a reader of the translation file knows about the element's location just by looking at the keys.
The following example is good practise: pages.profile.modals.contact.title="Contact Me".
10) Track and eliminate technical debt by using TODO comments.
Sometimes a feature needs to be delivered in a rush. If you have to make shortcuts in your code, add a // TODO comment for later cleanup. Your IDE likely has a feature to easily navigate TODOs. By regularly eliminating open TODO's, you keep the code base clean.